ELF has two related concepts for managing symbols in your programs. The first concept is the symbol binding. Global binding means the symbol is visible outside the file being built; local binding is the opposite and keeps the symbol local only (static) and weak is like global, but suggests that the symbol can be overridden.
$ cat syms.c static int local(void) { } int global(void) { } int __attribute__((weak)) weak(void) { } $ gcc -o syms -c syms.c $ readelf --syms ./syms Symbol table '.symtab' contains 10 entries: Num: Value Size Type Bind Vis Ndx Name ... 5: 00000000 8 FUNC LOCAL DEFAULT 1 local 8: 00000008 8 FUNC GLOBAL DEFAULT 1 global 9: 00000010 8 FUNC WEAK DEFAULT 1 weak ...
This is all well and good, but starts breaking down when you want to load many different modules and keep strict API's (such as, say, dynamic libraries!).
Consider that for two files to share a common function, the function must end up with a global visibility.
$ cat file1.c void common_but_not_part_of_api(void) { } $ cat file2.c extern void common_but_not_part_of_api(void); void api_function(void) { common_but_not_part_of_api(); } $ gcc -shared -fPIC -o library file1.c file2.c $ readelf --syms ./library Symbol table '.symtab' contains 60 entries: Num: Value Size Type Bind Vis Ndx Name ... 53: 00000424 29 FUNC GLOBAL DEFAULT 11 api_function 55: 0000041c 5 FUNC GLOBAL DEFAULT 11 common_but_not_part_of_api ...
In the example above, both the function we want exported (api_function) and the function we don't wish exported (common_but_not_part_of_api) end up with exactly the same attributes. Binding attributes are useful for the linker putting together object files; but aren't a complete solution.
To combat this, ELF provides for visibility attributes. Symbols can be default, protected, hidden or internal. Using these attributes, we can flag extra information for the dynamic loader so it can know which symbols are for public consumption, and which are for internal use only.
The most logical way to use this is to make all symbols by default hidden with -fvisibility=hidden and then "punch holes in the wall" for those symbols you want visible.
$ cat file1.c void common_but_not_part_of_api(void) { } $ cat file2.c extern void common_but_not_part_of_api(void); void __attribute__((visibility("default"))) api_function(void) { common_but_not_part_of_api(); } $ gcc -fvisibility=hidden -shared -fPIC -o library file1.c file2.c $ readelf --syms ./library Symbol table '.symtab' contains 60 entries: Num: Value Size Type Bind Vis Ndx Name 48: 000003cc 5 FUNC LOCAL HIDDEN 11 common_but_not_part_of_api 54: 000003d4 29 FUNC GLOBAL DEFAULT 11 api_function
Now the dynamic loader has enough information to distinguish between the two, and can stop any external access to common_but_not_part_of_api easily.
This extra information also has potential for performance improvements. Any time a symbol may be overridden, the compiler must generate a program lookup table (PLT) entry for the function so that the dynamic loader can re-direct the function call. The PLT is a trampoline which gets the correct address of the function being called (from the global offset table, GOT) and bounces the function call to the right place. An example should illustrate:
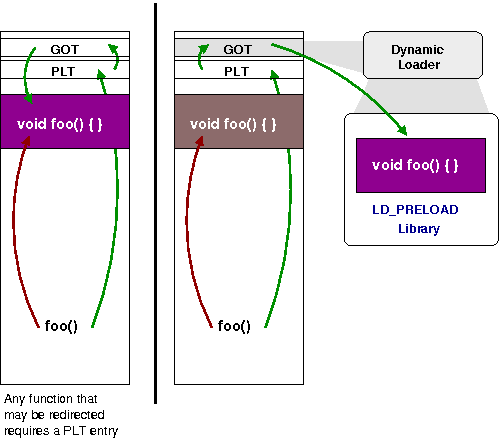
In the first example, there was not enough information to tell if the function would ever be able to be overridden, hence a PLT entry had to be created and the function called through it (disassemble it to see the details!). With correct symbol visibility attributes, there is enough information to know that common_but_not_part_of_api is never to be overridden, hence the PLT (and the associated costs of trampolining) can be avoided.
The internal attribute is even stricter; it says that this function will never be called from outside this module (for example, we might pass the address of common_but_not_part_of_api to anyone). This can lead to even better code, because on many architectures transitioning to another module might involve flipping global pointer registers or other similarly expensive operations.
So that's how symbol binding and visibility attributes can work together to get you the best performance possible from your program!